CELEBRITY RECOGNITION IN THE AVENGERS TRAILER WITH AI | AWS REKOGNITION | OPENCV | PYTHON | 2023
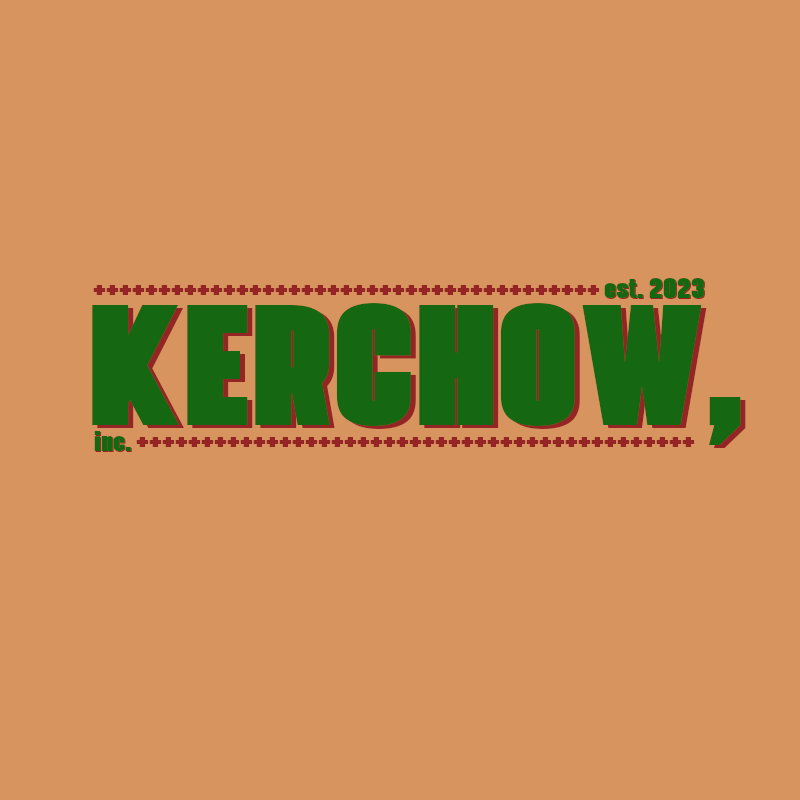
- Published on
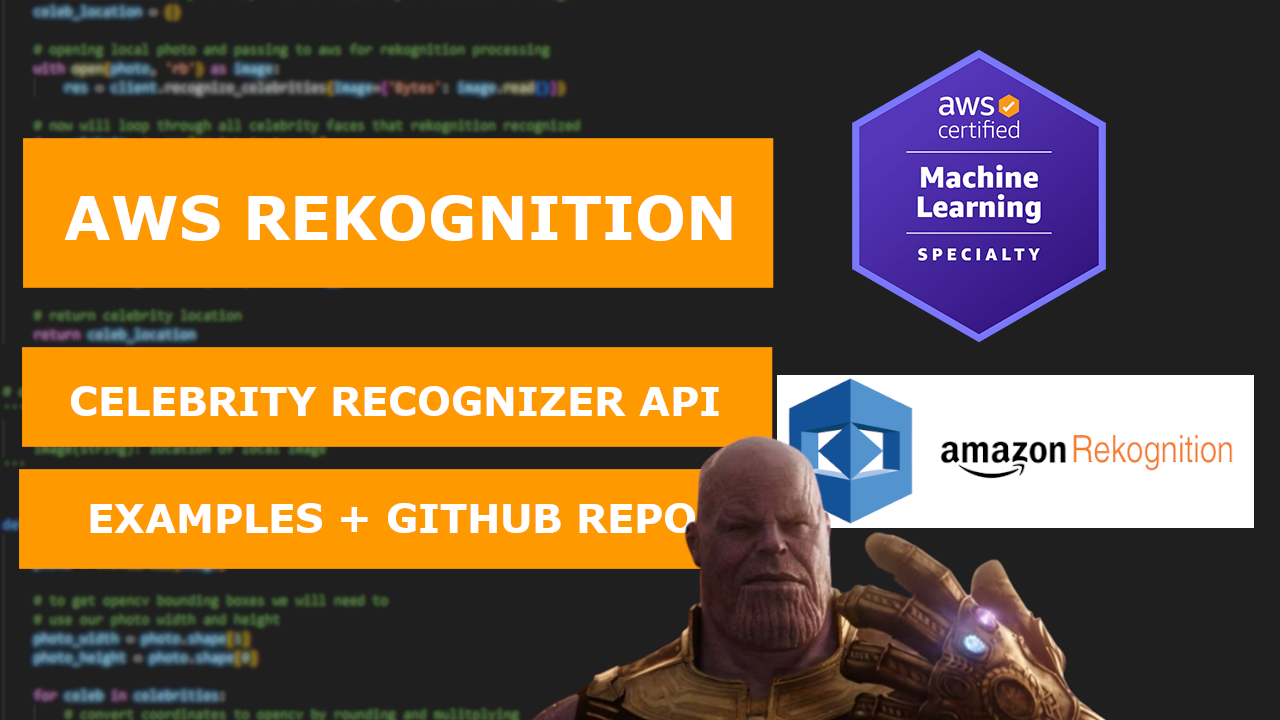
Write Up
This project focuses on AWS Rekognition, and IAM as well as OpenCV. The goal of the project was to display basic and advanced functionalities utilizing the celebrity recognizer api.
Utilizing AWS Rekognition the goal was accomplished within two different methods.
First being that celebrities were recognized and had bounding boxes drawn around each celebrities face with name below.
Second was using an Avengers trailer to replicate Amazon Prime X-Ray to show what celebrities are currently on screen and to overlay a picture and name of the celebrities onto the Avengers trailer.
The GitHub for this project is here: https://github.com/kerchow-inc/Celebrity-Rekognition-Trailer
Important Functions
The main functions to discuss are "recognize_celebrities" and "celebrity_bounding_boxes".
recognize_celebrities - This function shows how to interact with the recognize celebrities api as well as sending the image as bytes to the api. Also happening in the function is the process of creating a dictionary that will store the bounding boxes of each celebrity that was recognized in the photo.
celebrity_bounding_boxes - As in the name this function draws bounding boxes as well as inserting the name onto the original photo. When creating the bounding boxes however, they must first be converted to OpenCV bounding box coordinates from AWS coordinates which is seen in the function. Once all bounding boxes of each celebrity are drawn then the photo will display and wait for the input of "0" from the user to close the window.
# celebrity recognition
'''
photo(string): local image location
'''
def recognize_celebrities(photo):
# create a dictionary to keep track of celebrity and location in image
celeb_location = {}
# opening local photo and passing to aws for rekognition processing
with open(photo, 'rb') as image:
res = client.recognize_celebrities(Image={'Bytes': image.read()})
# now will loop through all celebrity faces that rekognition recognized
for celebrity in res['CelebrityFaces']:
# name of recognized celebrity
name = celebrity['Name']
# location of celebrities face
bounding_box = celebrity['Face']['BoundingBox']
if name not in celeb_location:
celeb_location[name] = bounding_box
# return celebrity location
return celeb_location
# draw celebrity bounding boxes
'''
celebrities(dictionary): {celebrity_name: bounding_box_dictionary}
image(string): location of local image
'''
def celebrity_bounding_boxes(celebrities, image):
# get local image loaded
photo = cv2.imread(image)
# to get opencv bounding boxes we will need to
# use our photo width and height
photo_width = photo.shape[1]
photo_height = photo.shape[0]
for celeb in celebrities:
# convert coordinates to opencv by rounding and mulitplying
# by photo width and height
left = round(celebrities[celeb]['Left']*photo_width)
top = round(celebrities[celeb]['Top']*photo_height)
width = round(celebrities[celeb]['Width']*photo_width)
height = round(celebrities[celeb]['Height']*photo_height)
# draw our bounding box onto the photo
cv2.rectangle(photo, (left, top),
(left + width, top + height), (255, 255, 0), 4)
# insert the celeb name at the bottom left corner
cv2.putText(photo, celeb, (left+25, top + height+25),
cv2.FONT_HERSHEY_COMPLEX, 1, (255, 255, 0), 2)
# show our photo
cv2.imshow('Celebrity Rekognition', photo)
# pressing 0 on keyboard will close image
cv2.waitKey(0)
cv2.destroyAllWindows()
Watch on YouTube
Want to get a more indepth reasoning behind why certain code is written or missing explanations of the rest of the code?
Watch the video below!
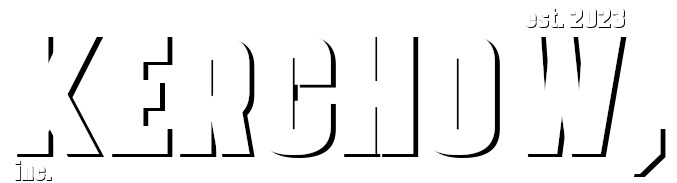